It is a best practise to write neat and clean codes. Every companies expect developers to write neat and standard codes. But the big question is how to improve our codes?
Writing a neat and clean code is not a big deal. You just need a few things to keep in mind and that’s it. In this article we will look at some great ways to improve your Angular code and believe me, your code will become a standard code and it will pass any code analysis tool easily.
Table of Contents
7 Best Ways to improve your Angular code
- Avoid using
Any
Data Type: you can useString
,Number
, or aModel
(in case of Object) Data Type instead - Remove usage of
var
: uselet
,const
instead - Create and consume Interfaces: these interfaces can be used as a
Model
Data Type - Avoid keeping any unnecessary code on page: remove unused variables / codes if there is any
- Use lazy loaded child components
- Create private / public methods as per requirement
- Avoid hardcoded values: use constants / enums
Let’s understand each of them one by one.
Avoid using Any Data Type
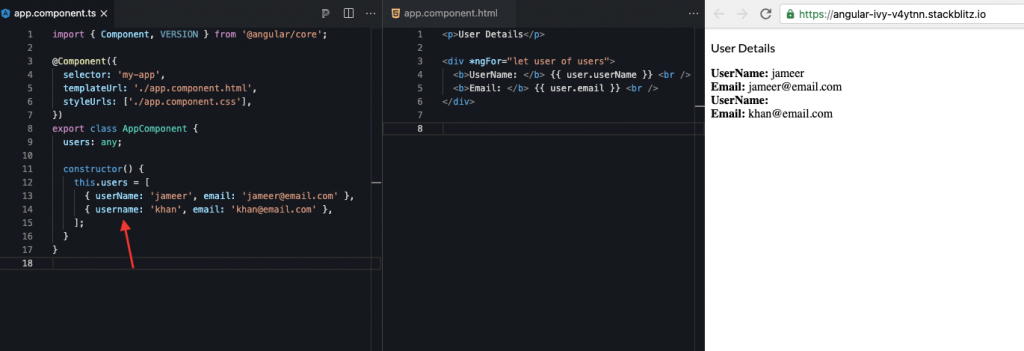
I have declared an array variable users
with any
Data Type. The variable is filled with some objects in constructor
to display on page.
The common problem having any
in your code is the VS Code doesn’t complain you about the field typos. If you notice in code, I have written username
instead of userName
but still the VS Code is not complaining about the error and due to typo, no data is shown on browser for the wrong field (check in above screenshot).
Here is what happens when you have a proper Model Data Type.
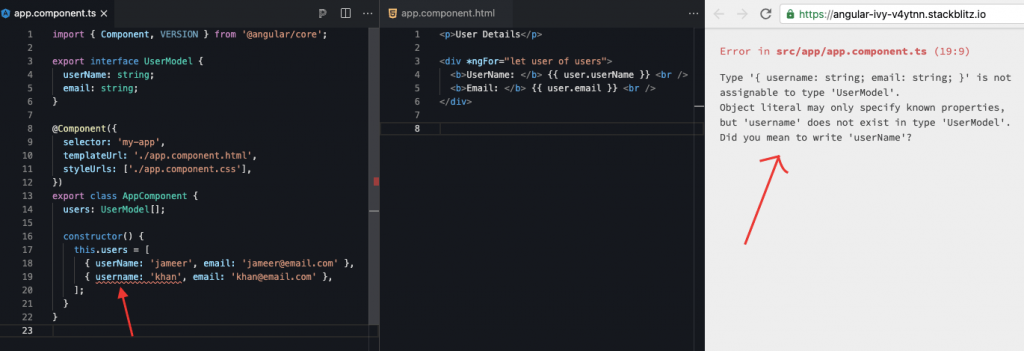
You can clearly see that it throws error when there is a Model Data Type.
The above example clearly states to use the proper data type instead of any
.
Remove usage of var
There should not be any use of var
in the project. It is a globally declaration variable. The recommended way to declare a variable is let
and const
.
Both are block scoped variables
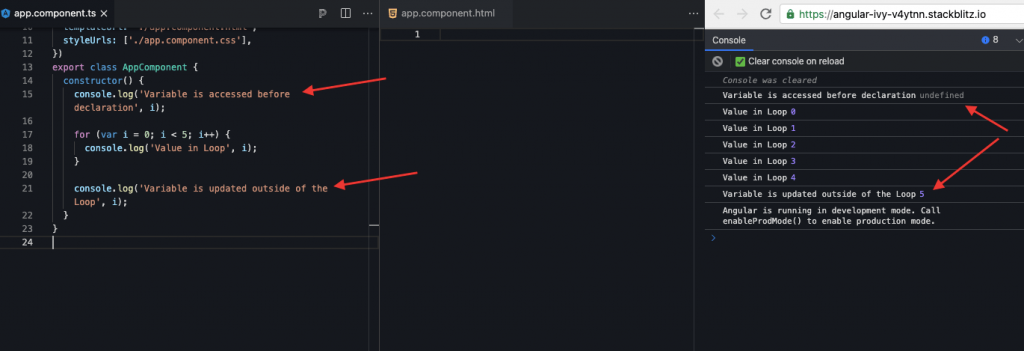
There are two common problems of using var
:
- Variable can be accessed even before its declaration (check it in screenshot above)
- Variable value is updated even outside of the block (check it in screenshot above)
Create and consume Interfaces
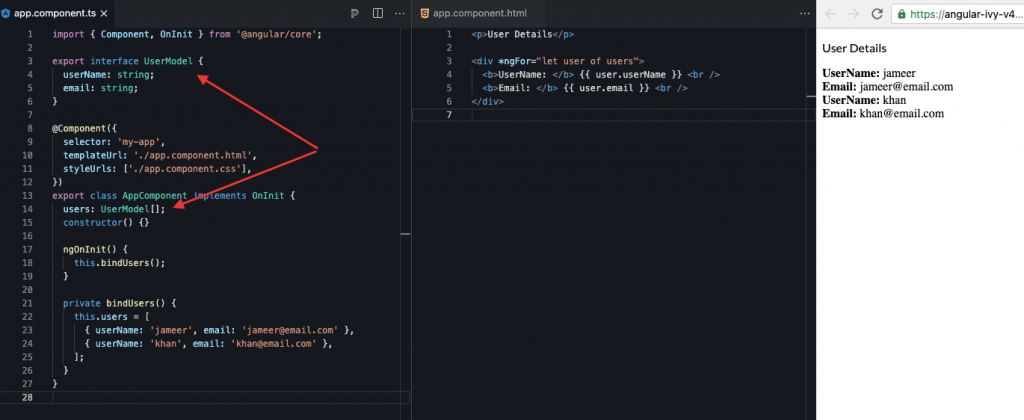
You should have interface to work with any of your Objects. You should avoid using any
data type as already mentioned in first point.
In the above code I have created an interface UserModel
and consumed that while declaring a variable users
. It helps you with binding its properties in template files easily as well as plot any typo errors.
Avoid keeping any unnecessary code on page
You should keep optimizing your codes again and again so that you will not feel a big task to optimize it. Remove any unnecessary unused variables, codes, scripts, links, css, classes, functions etc anything from your code.
Use lazy loaded child components
Lazy loading is a great way to achieve a good performance from a website. It loads only the required component at a time.
For example you are accessing Student Module and lazy loading is already integrated there, so it will load only the required components, css, scripts related to Student Module.
Create private / public methods as per requirement
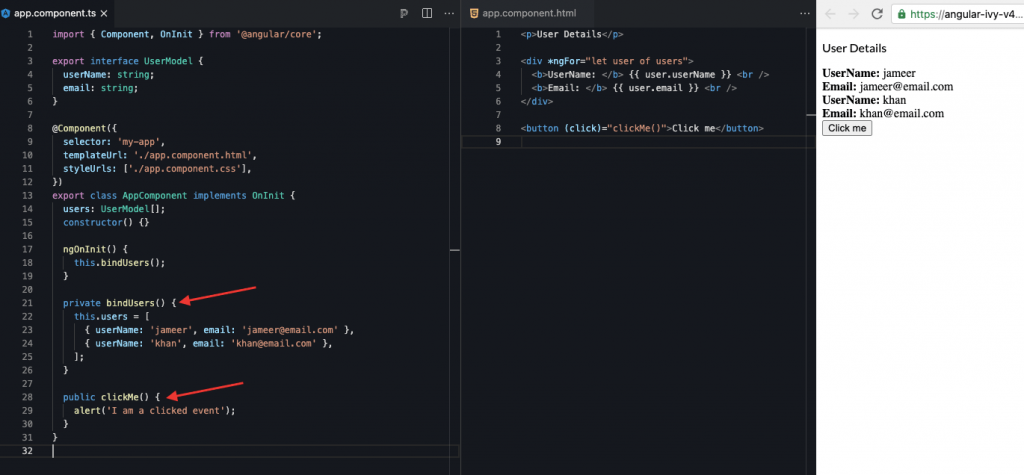
I have used two methods- bindUsers()
and clickMe()
One of them is called at ngOnInit()
which is a life cycle of Angular and another of them is called from the template on button click.
bindUsers()
can be private
because this method doesn’t need accessibility from template or from any other file.
But clickMe()
should be public
because that requires accessibility from its template. In case of public
we do not need to specify it. The method is by default public if there is no access modifier present.
Avoid hardcoded values
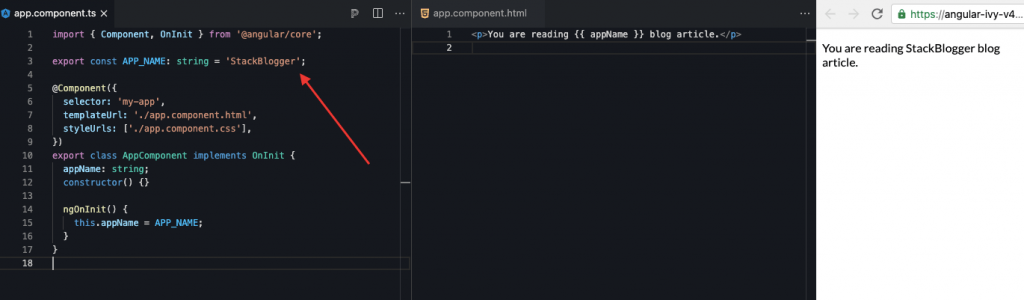
Avoid any hardcoded values in code. Create a separate Constant
or Enum
file and put your values in those files and consume in components.
Above is an example of using a constant variable APP_NAME
and consuming it on component.
What’s next
Well in this article I have covered 7 best ways to improve your angular codes. I believe you should have an idea about how you can write a neat and clean codes. I would recommend you to check 5 most used VS Code extensions for Angular Developers.
15 thoughts on “7 Best Ways To Improve Angular Code (2022)”