Website development is one thing and optimization is another. Optimization becomes difficult with time to time as application grows. To avoid that difficulty, you should keep in mind about some points at the time of creating the application. In this article we will talk about some ways to optimize your Angular project that will eventually help you with scaling the application.
If you are looking for some ways to improve your Angular coding standards then I would highly recommend this article: 7 Best Ways to Improve Angular Code (opens in a new tab).
Let’s start with the article.
Table of Contents
5 Best Ways to Optimize Angular for Scaling
- Separation of Concern: a well defined folder structure
- API Data Management: proper use of observables and subscriptions
- TrackBy function: re-render only updated records
- PWA: use of progressive web apps
- Change Detection: run change detection explicitly
I will go through each of the mentioned points above and explain with example.
Separation of Concern
It is a concept of separating business and presentation logics. A detailed case study on why separation of concern is required, is mentioned in an article at Medium by Georgi Parlakov: The Page Pattern.
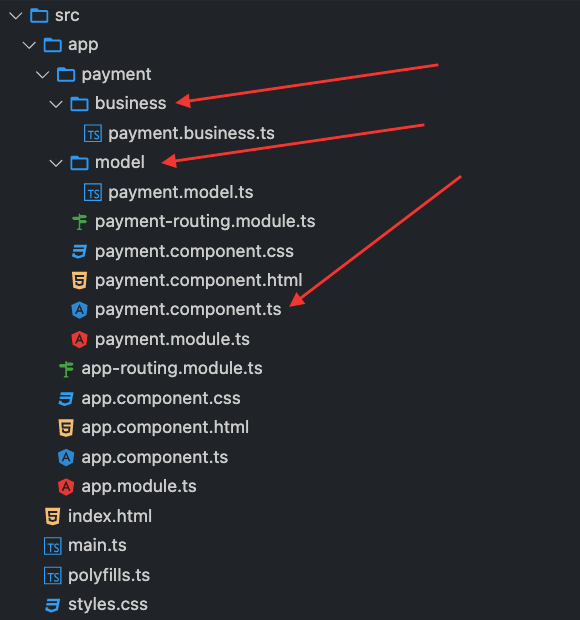
Above screenshot clearly shows that there is a component
file, a model
file and a business
file for Payment page. It is recommended to use models for objects you are dealing with.
Business logics are the part of your code which interacts with HTTP Service and component events (user events like add / update / delete etc).
Presentation logics are the part of your code which have variables, events and any UI related modification codes.
Keeping presentation and business logic separate is required because they will help you in scaling the application. There will not be code mix ups between business and presentation as functionality grows.
Benefits of using Separation of Concern:
- Code reusability
- Easy to understand (in case new developer works on it)
- Easier to implement a new functionality
- Easy to write Unit Testing
API Data Management
RxJS provides many operators to use while working with Observables / API Responses. A proper use of RxJS operators will help improve the performance of application instead of slowing down.
For example: you have to call User API which gives you User Id and based on the ID, you have to call User Address API which will give you address detail of the particular user. Now instead of calling the User Detail API in subscribe, call it from concatMap
operator.
Use async pipes instead of subscription. Subscription of observables will cause problem with OnPush
detection.
Async pipe handles the subscriptions automatically so no need to manually unsubscribe the observables.
UnSubscribe the subscriptions in ngOnDestroy
so that there is no memory leakage.
An easy way to unsubscribe subscriptions is add all the subscriptions in the subscription array and unsubscribe the subscription at once in ngOnDestroy
.
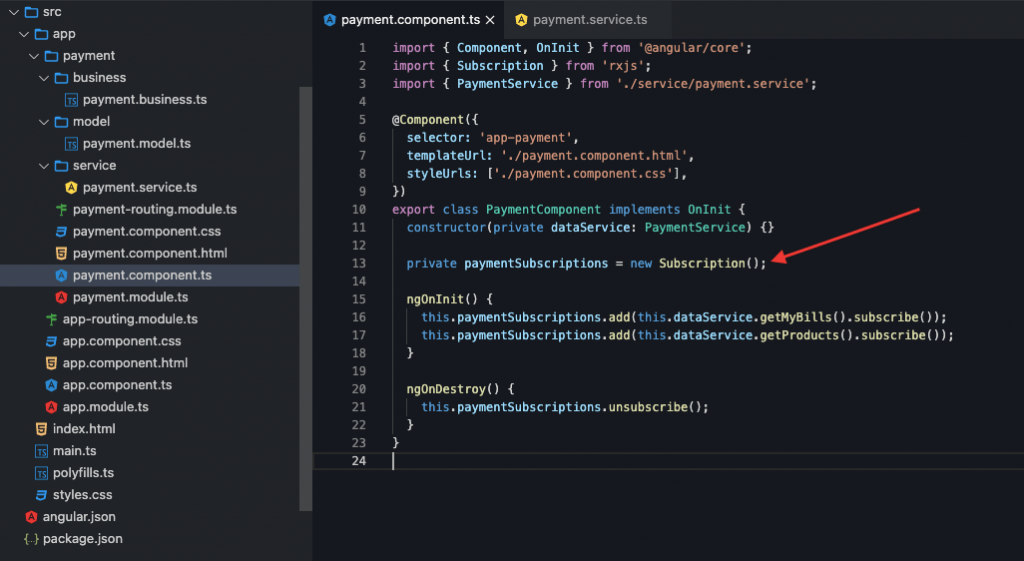
TrackBy Function
*ngFor
by default re-render all the elements present in the array. It consumes browser memory when you have many for loops on the page or even one for loop with large data.
There is no need to re-render the items which are not updated by the API. *ngFor
keeps track on the updated items and re-renders only the updated one. It clearly improves your application speed.
Here is how to use trackBy
function in *ngFor
app.component.ts
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-main', templateUrl: './app.component.html', styleUrls: ['./app.component.css'], }) export class AppComponent implements OnInit { items = [{ name: 'Jameer', email: 'jameer@email.com' }]; constructor() {} ngOnInit() {} identify(index, item) { return item.email; } } |
app.component.html
1 2 3 | <div *ngfor="let item of items; trackBy: identify"> {{ item.name }} </div> |
PWA
Progressive Web Apps are web apps that use services workers, manifests and other web components to give user a best experience even on the low speed internet.
You should use PWA if your application does extensive api calls, complex calculations, image filtering etc.
Benefits of using PWA:
- Background sync
- Offline support
- Rich caching
- Push notifications
- and many more
Follow the steps to integrate PWA in Angular app:
Add PWA
ng add @angular/pwa --project <your angular project name>
In case you don’t know what is your project name: open package.json and check the “name” field.
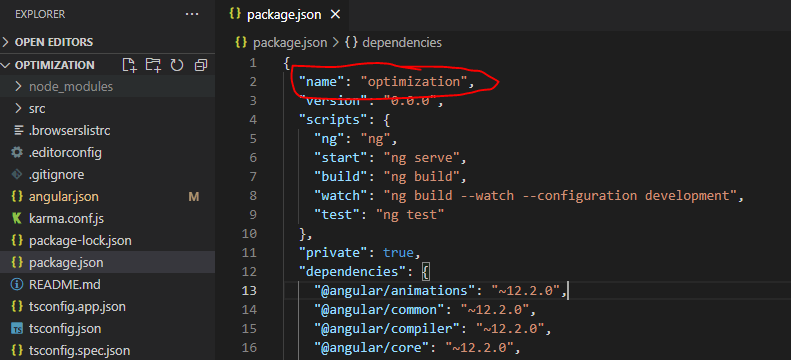
Running above command will automatically add the required files to setup a service worker along with updating index.html
file.
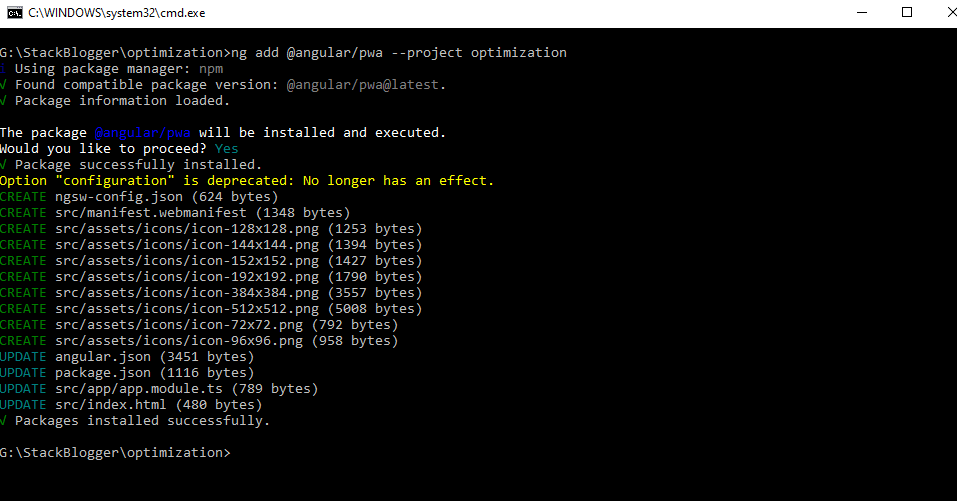
That’s it! The service worker is integrated and the app is ready to test.
How to test if PWA is integrated successfully and working
First of all build your angular application.
ng b --prod
We would need to install a Node.Js package http-server
in order to test the service worker. Install http-server
with below command:
npm install --global http-server
Once the http-server
is installed, run below command:
http-server -p 8080 -c-1 dist/<your angular project name>
The application will start running at port 8080. Open http://localhost:8080 in your browser to view the running app.
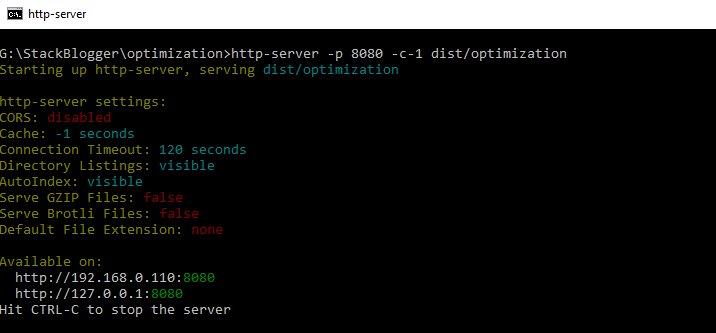
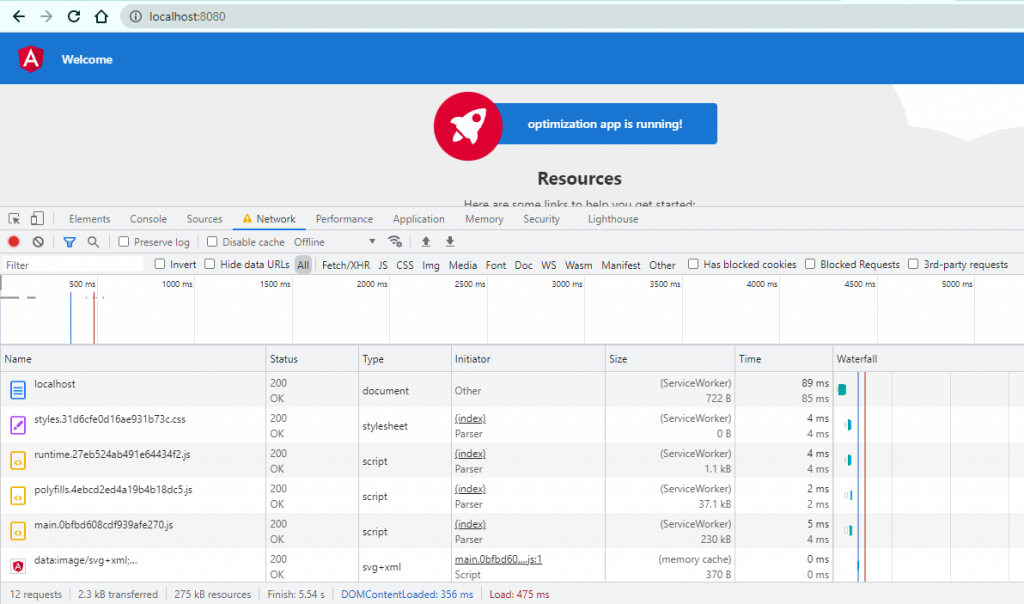
You did it! Go to Network -> Click on No Throttling dropdown -> Choose Offline -> Reload the page. You will notice your files are being loaded from Service Worker.
Change Detection
Change detection is something which is run by Angular by default. But there is a good news, we can run it explicitly as well.
It is a powerful mechanism to improve performance. It detects new entries and update on DOM. The changed data gets displayed on the page.
It works fine for smaller applications. The problem comes when the application is large. It goes to main component and consistently checks for the changes in sub components and update them.
We can optimize it to check the changes only when required.
These three strategies a developer should use to improve the performance.
- OnPush & Immutability
- Pipes instead of methods
- Detach change detection
Conclusion
In this article I have covered 5 best ways to optimize an Angular application that will eventually help in better scaling of application.
If you are looking out for some best basic ways to standardized your angular code, I would recommend check this article: 7 Best Ways to Improve Angular Code.
Also Read: 5 Most Used VS Code Extensions for Angular Developers